Databinding using an ObjectDataSource control in Relatude
Sometimes an ObjectDataSource can be a good method to bind data to databound controls. This shows an example on how to do it with Relatude.
About the example
In the example, I'm using the Relatude Framework starter site, and I'm fetching a list of all the Articles in the system. I'm using a GridView to display the data. First, we'll take a look at the ASPX file:
<asp:GridView runat="server" ID="gridView2" AutoGenerateColumns="false" DataKeyNames="NodeId" AllowPaging="true" PageSize="20" DataSourceID="wafODS">
<Columns>
<asp:BoundField DataField="Name" HeaderText="Name" />
<asp:BoundField DataField="CreateDate" HeaderText="CreateDate" DataFormatString="{0:dd.MM.yy HH:mm}" />
</Columns>
</asp:GridView>
<asp:ObjectDataSource ID="wafODS" EnablePaging="true" runat="server" SelectCountMethod="GetArticlesCount" SelectMethod="GetArticles" TypeName="ArticleObjectDataSource"></asp:ObjectDataSource>
As you can see above, there is very little code. The GridView specifies the ID of the ObjectDataSource control. The ObjectDataSource control specifies two methods: GetArticlesCount and GetArticles. The TypeName is the name of the class that the ObjectDataSource control loads data from. Let's look at that class:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using WAF.Engine.Content.Native;
using WAF.Presentation.Web;
using WAF.Engine.Query.Native;
using WAF.Engine.Query;
public class ArticleObjectDataSource
{
public ArticleObjectDataSource(){
}
public List<ArticleBase> GetArticles(int startRowIndex, int maximumRows) {
int pageSize = maximumRows;
int pageIndex = 0;
int totalCount = 0;
if (startRowIndex > 0) {
pageIndex = (int)Math.Round(((double)startRowIndex / (double)pageSize));
}
return WAFContext.Session.Query<ArticleBase>().OrderBy(AqlArticleBase.PublishDate, true).Execute(pageIndex, pageSize, out totalCount);
}
public int GetArticlesCount() {
AqlQuery q = WAFContext.Session.CreateQuery();
AqlAliasArticleBase aliasArticleBase = new AqlAliasArticleBase();
AqlResultInteger count = q.Select(Aql.Count(aliasArticleBase.NodeId));
q.From(aliasArticleBase);
AqlResultSet rs = q.Execute();
int c = 0;
while (rs.Read()) {
c = count.Value;
}
return c;
}
}
The results
The code above will result in this being displayed in the template:
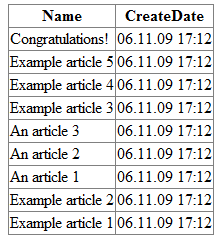