Using coupon codes in the e-commerce module
The e-commerce module supports coupon codes. In this tutorial I'll show you how to set it up.
Background
Coupon codes can be defined as "is a code, consisting of letters or numbers that consumers can enter into a promotional box on a site's shopping cart checkout page to obtain a discount on their purchase, such as a percentage off purchase, free shipping, or other discount." From Webopeia
In the Relatude e-commerce module, coupon codes are part of the discount system. All discounts can be used as a coupon code. This is done by adding a coupon code to the "Coupon code" property on all discounts. If a coupon code is added to a discount, the discount isn't applied automatically to an order anymore, even if all the other criteria are fullfilled.
A coupon code has to be applied manually to an order. The interface to allow the user to do this is something that has to be added to a template, normally the shopping cart template. Below, I'll describe in detail how to do it.
Adding the coupon code to a discount
It is very simple to use coupon codes with discounts. Just add a code to the "Coupon code" property and save:
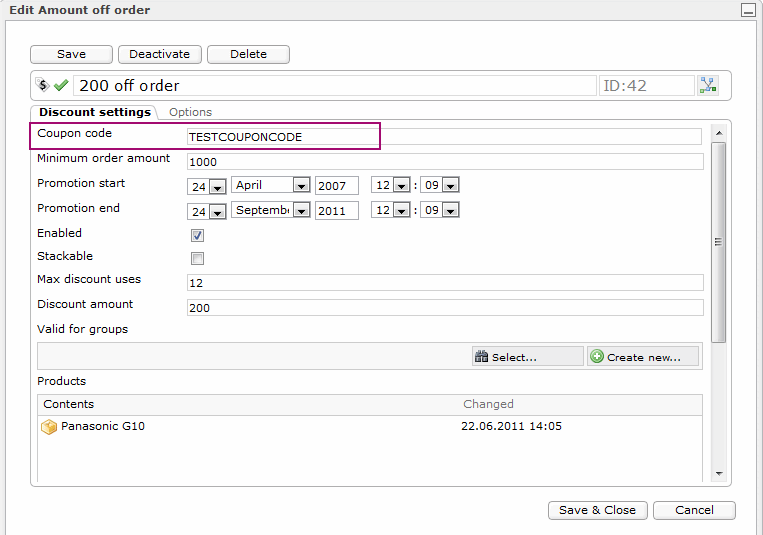
As you can see above, you fill out the rest of the discount properties as normal. The only thing you change when adding a coupon code is that you force your customer to do something actively to get the discount.
Implementing it in a template
To make it possible for the user to apply the discount, you have to create a UI of some sort that allows them to apply discounts to an order. There isn't a gui for this included in Relatude, but at the most basic level, it's only a few lines of code.
In this example, I'm going to add a textbox, a literal and a button to the shopping cart template. The code looks like this. The textbox will let the users type in the coupon code, the literal will notify the user if the coupon was applied successfully. This is how the code looks like:
<asp:Literal runat="server" ID="litResult" EnableViewState="false"></asp:Literal>
<br />
<asp:TextBox runat="server" ID="txtCouponCode"></asp:TextBox><asp:Button runat="server" ID="btnAddCoupon" OnClick="btnAddCoupon_Click" Text="Add coupon" />
There's nothing fancy in the aspx page. Now, let's look at the code in the button click event:
protected void btnAddCoupon_Click(object sender, EventArgs e) {
Order order = WAFShopHelper.GetCurrentOrder(Request, Response);
if (order != null) {
CouponCodeAddResult addResult = order.AddCouponCode(txtCouponCode.Text.Trim());
if (addResult.Success) {
order.UpdateChanges();
}
litResult.Text = "<b><span style=\"color:red;\">" + addResult.Message + "</span></b>";
}
}
How it looks
There is basically no restrictions how you want the UI of the coupon functionality to look, but this is how it looks in this example:
The coupon textbox before we do anything:
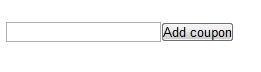
You can see we have no discounts applied to the current shopping cart before we apply the coupon code:
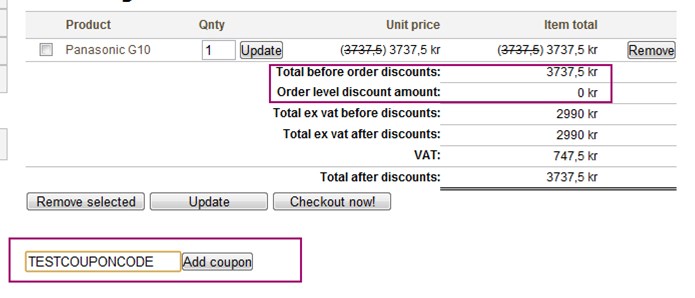
The "Order level discount amount" is 0. You can also see above that we have typed in the coupon code, but we haven't clicked the button yet. When we do, this is the result:
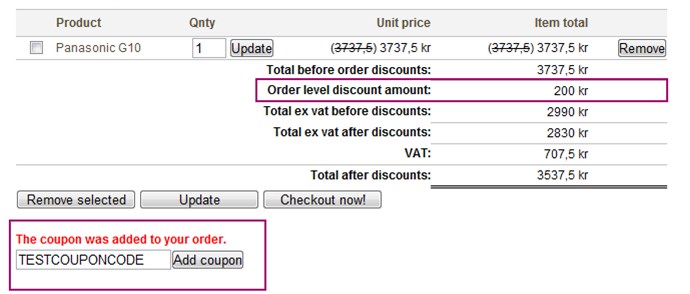
A few things to notice:
- The "Order level discount amount" has been changed to 200.
- The cart price has been changed, and it's 200 lower than it used to be.
- The user got a message back saying the coupon was added to the order.
But if the coupon code isn't valid anymore, or if the user types something wrong, this is how he is notified:
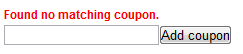