Databinding using nested repeaters with Relatude
You often get into situations where you want to create lists of nodes, and for each list item you want to add a list of sub items or related content. Nested repeaters a common solution to those situations.
In this example, I'll print all the direct children of the site in one repeater. For each child, I'll databind the child's children to the nested repeater. The code in the aspx file:
<asp:Repeater runat="server" ID="repChildren" OnItemDataBound="repChildren_ItemDataBound">
<HeaderTemplate>
<ul>
</HeaderTemplate>
<ItemTemplate>
<li>
<asp:Literal runat="server" ID="litName" Text='<%# Eval("Name") %>' EnableViewState="false"></asp:Literal>
<asp:Repeater runat="server" ID="repSubChildren">
<HeaderTemplate>
<ul>
</HeaderTemplate>
<ItemTemplate>
<li>
<asp:Literal runat="server" ID="litName" Text='<%# Eval("Name") %>' EnableViewState="false"></asp:Literal>
</li>
</ItemTemplate>
<FooterTemplate>
</ul>
</FooterTemplate>
</asp:Repeater>
</li>
</ItemTemplate>
<FooterTemplate>
</ul>
</FooterTemplate>
</asp:Repeater>
The code above is very simple. There is an outer repeater, which has one event method defined: OnItemDataBound. Apart from that, the only thing to notice is that the ItemTemplate contains another Repeater.
In order to bind data to the outer repeater, I'll fetch the children of the current site:
Site site = WAFContext.Session.GetContent<Site>(WAFContext.Session.SiteId);
repChildren.DataSource = site.Children.Get();
repChildren.DataBind();
We also need to do something to bind the children of the children to the nested repeater. To do that, we use the OnItemDataBound event. In that event we got access to all the controls in the templates, as well as the data item itself. This allows us to do this:
protected void repChildren_ItemDataBound(object sender, RepeaterItemEventArgs e) {
Repeater rep = (Repeater)e.Item.FindControl("repSubChildren");
HierarchicalContent hc = (HierarchicalContent)e.Item.DataItem;
if (rep != null) {
rep.DataSource = hc.Children.Get();
rep.DataBind();
}
}
The result
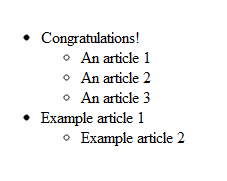